Introduction
Within the dynamic panorama of machine studying, synthesizing two potent methods has given rise to a flexible mannequin often called Adversarial Autoencoders (AAEs). Seamlessly mixing the options of autoencoders and Generative Adversarial Networks (GANs), AAEs have emerged as a robust instrument for information era, illustration studying, and past. This text explores the essence of AAEs, their structure, coaching course of, and functions and supplies a hands-on Python code instance for an enriched understanding.
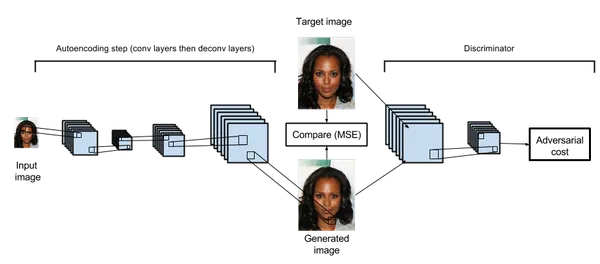
This text was revealed as part of the Knowledge Science Blogathon.
Understanding Autoencoders
Autoencoders, the muse of AAEs, are neural community constructions designed for information compression, dimensionality discount, and have extraction. The structure consists of an encoder that maps enter information right into a latent house illustration, adopted by a decoder that reconstructs the unique information from this decreased illustration. Autoencoders have been instrumental in varied fields, together with picture denoising, anomaly detection, and latent house visualization.
Autoencoders, a elementary class of neural networks, can extract significant options from information whereas enabling environment friendly dimensionality discount. Comprising two fundamental parts, an encoder compresses enter information right into a lower-dimensional latent illustration, whereas the decoder reconstructs the unique enter from this compressed kind. Autoencoders serve varied functions, together with denoising, anomaly detection, and illustration studying. Their capability to seize important information traits makes them a flexible instrument for duties throughout domains comparable to picture processing, pure language processing, and extra. By studying compact but informative representations, autoencoders provide invaluable insights into the underlying constructions of complicated datasets.
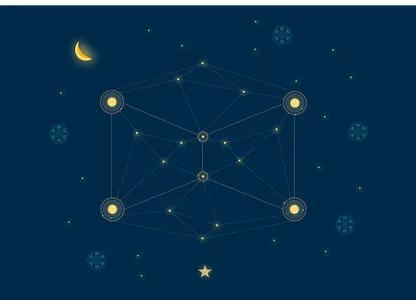
Introducing Adversarial Autoencoders
Adversarial Autoencoders (AAEs) are a outstanding fusion of autoencoders and Generative Adversarial Networks (GANs), innovatively combining their strengths. This hybrid mannequin introduces an encoder-decoder structure the place the encoder maps enter information right into a latent house, and the decoder reconstructs it. The distinctive ingredient of AAEs lies in integrating adversarial coaching, the place a discriminator critiques the standard of generated information samples. This adversarial interplay between the generator and discriminator refines the latent house, fostering high-quality information era.
AAEs discover various functions in information synthesis, anomaly detection, and unsupervised studying, yielding strong latent representations. Their versatility presents promising avenues in varied domains, comparable to picture synthesis, textual content era, and so on. AAEs have garnered consideration for his or her potential to boost generative fashions and contribute to the development of synthetic intelligence.
Adversarial Autoencoders, the results of integrating GANs with autoencoders, add an progressive dimension to generative modeling. By combining the latent house exploration of autoencoders with the adversarial coaching mechanism of GANs, AAEs steadiness the advantages of each worlds. This synergy ends in enhanced information era and extra significant representations within the latent house.
AAE Structure
The architectural blueprint of AAEs revolves round three pivotal parts: the encoder, the generator, and the discriminator. The encoder condenses enter information right into a compressed illustration within the latent house whereas the generator reconstructs the unique information from these compressed representations. The discriminator introduces the adversarial facet, aiming to distinguish between precise and generated information samples.
Coaching AAEs
The coaching of AAEs is an iterative dance of three gamers: the encoder, the generator, and the discriminator. The encoder and generator collaborate to attenuate the reconstruction error, guaranteeing that the generated information resembles the unique enter. Concurrently, the discriminator hones its expertise in distinguishing between actual and generated information. This adversarial interplay results in a refined latent house and improved information era high quality.
Functions of AAEs
The flexibility of AAEs is exemplified by means of a spectrum of functions. AAEs shine in information era duties, able to producing sensible samples in domains comparable to pictures, textual content, and extra. Their anomaly detection prowess finds utility in figuring out irregularities inside datasets. Moreover, AAEs are adept at unsupervised illustration studying, aiding function extraction and switch studying.
Anomaly Detection and Knowledge Denoising: AAEs’ latent house regularization empowers them to filter out noise and anomalies in information, rendering them a strong selection for information denoising and anomaly detection duties.
Type Switch and Knowledge Transformation: By manipulating latent house vectors, AAEs allow model switch between inputs, seamlessly morphing pictures and producing various variations of the identical content material.
Semi-Supervised Studying: AAEs can harness labeled and unlabeled information to enhance supervised studying duties, bridging the hole between supervised and unsupervised approaches.
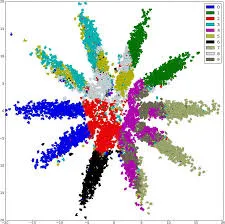
Implementing an Adversarial Autoencoder
To offer a sensible understanding of AAEs, let’s delve right into a Python implementation utilizing TensorFlow. On this instance, we’ll concentrate on information denoising, showcasing how AAEs can excel in reconstructing clear information from noisy enter.
(Word: Guarantee you’ve gotten TensorFlow and related dependencies put in earlier than working the code under.)
import tensorflow as tf
from tensorflow.keras.layers import Enter, Dense, Flatten, Reshape
from tensorflow.keras.fashions import Mannequin
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.losses import MeanSquaredError
from tensorflow.keras.datasets import mnist
import numpy as np
# Outline the structure of the Adversarial Autoencoder
def build_adversarial_autoencoder(input_dim, latent_dim):
input_layer = Enter(form=(input_dim,))
# Encoder
encoder = Dense(128, activation='relu')(input_layer)
encoder = Dense(latent_dim, activation='relu')(encoder)
# Decoder
decoder = Dense(128, activation='relu')(encoder)
decoder = Dense(input_dim, activation='sigmoid')(decoder)
# Construct and compile the autoencoder
autoencoder = Mannequin(input_layer, decoder)
autoencoder.compile(optimizer=Adam(), loss=MeanSquaredError())
# Construct and compile the adversary
adversary = Mannequin(input_layer, encoded)
adversary.compile(optimizer=Adam(), loss="binary_crossentropy")
return autoencoder, adversary
# Load and preprocess MNIST dataset
(input_train, _), (input_test, _) = mnist.load_data()
input_train = input_train.astype('float32') / 255.0
input_test = input_test.astype('float32') / 255.0
input_train = input_train.reshape((len(input_train), np.prod(input_train.form[1:])))
input_test = input_test.reshape((len(input_test), np.prod(input_test.form[1:])))
# Outline AAE parameters
input_dim = 784
latent_dim = 32
# Construct and compile the AAE
autoencoder, adversary = build_adversarial_autoencoder(input_dim, latent_dim)
# Prepare the AAE
autoencoder.match(input_train, input_train,
epochs=50,
batch_size=256,
shuffle=True,
validation_data=(input_test, input_test))
# Generate denoised pictures
denoised_images = autoencoder.predict(input_test)
Hyperparameter Tuning
Hyperparameter tuning is important to coaching any machine studying mannequin, together with Adversarial Autoencoders (AAEs). Hyperparameters are settings that decide the conduct of the mannequin throughout coaching. Correctly tuning these hyperparameters can enormously affect the generated samples’ convergence velocity, stability, and high quality. Some vital hyperparameters embrace Studying Fee, Coaching Epochs, Batch dimension, Latent Dimension, Regularization Energy, and so on. For simplicity, we will probably be tuning two hyperparameters right here: variety of coaching epochs and batch dimension.
# Hyperparameter Tuning
epochs = 50
batch_size = 256
# Prepare the AAE
autoencoder.match(input_train, input_train,
epochs=epochs,
batch_size=batch_size,
shuffle=True,
validation_data=(input_test, input_test))
# Generate denoised pictures
denoised_images = autoencoder.predict(input_test)
Analysis Metrics
Evaluating the standard of generated information from AAEs is essential to make sure the mannequin produces significant outcomes. Listed below are just a few analysis metrics generally used:
- Reconstruction Loss: This measures how effectively the generated samples will be reconstructed again to the unique information. Decrease reconstruction loss signifies higher high quality of generated samples.
- Inception Rating: Inception Rating measures the standard and variety of generated pictures. It makes use of an auxiliary classifier educated on actual information to judge the generated samples. Increased Inception Scores point out higher range and high quality.
- Frechet Inception Distance (FID): FID calculates the gap between function distributions of actual and generated information within the Inception mannequin’s function house. Decrease FID values point out that the generated samples are nearer to actual information relating to statistics.
- Precision and Recall of Generated Knowledge: Metrics from the sector of knowledge retrieval will also be utilized to generated information. Precision measures the proportion of top of the range generated samples, whereas recall measures the proportion of high-quality actual samples which might be efficiently generated.
- Visible Inspection: Whereas not a quantitative metric, visually inspecting the generated samples can present insights into their high quality and variety.
# Analysis Metrics
def compute_inception_score(pictures, inception_model, num_splits=10):
scores = []
splits = np.array_split(pictures, num_splits)
for cut up in splits:
split_scores = []
for img in cut up:
img = img.reshape((1, 28, 28, 1))
img = np.repeat(img, 3, axis=-1)
img = preprocess_input(img)
pred = inception_model.predict(img)
split_scores.append(pred)
split_scores = np.vstack(split_scores)
p_y = np.imply(split_scores, axis=0)
kl_scores = split_scores * (np.log(split_scores) - np.log(p_y))
kl_divergence = np.imply(np.sum(kl_scores, axis=1))
inception_score = np.exp(kl_divergence)
scores.append(inception_score)
return np.imply(scores), np.std(scores)
Conclusion
As Generative AI continues to captivate researchers and practitioners alike, Adversarial Autoencoders emerge as distinct and versatile members of the generative household. By marrying the reconstruction prowess of autoencoders with the adversarial dynamics of GANs, AAEs navigate the fragile dance of knowledge era and latent house regularization. Their capability to denoise, remodel types, and harness the power of labeled and unlabeled information renders them an important toolset within the arsenal of artistic AI. As this journey concludes, Adversarial Autoencoders beckon us to unlock new dimensions in generative AI and forge a path towards information synthesis that seamlessly marries management and innovation.
- Adversarial Autoencoders (AAEs) merge autoencoders and adversarial networks to reconstruct information and regularize the latent house.
- AAEs discover functions in anomaly detection, information denoising, model switch, and semi-supervised studying.
- The adversarial element in AAEs introduces a critic community that enforces latent house distribution adherence, balancing creativity and management.
- Implementation of AAEs requires a mixture of deep studying ideas, adversarial coaching, and autoencoder structure.
- Exploring the panorama of Adversarial Autoencoders supplies a singular perspective on generative AI, opening doorways to novel information transformation and regularization paradigms.
Often Requested Questions
A1: AAEs introduce adversarial coaching, enhancing their information era capabilities and latent house representations.
A2: The discriminator in AAEs sharpens the latent house by distinguishing between real and generated information, fostering improved information era.
A3: AAEs excel in anomaly detection, recognizing deviations from regular information patterns.
A4: Researchers have delved into conditional AAEs and domain-specific diversifications, tailoring AAEs to specific duties.
The media proven on this article will not be owned by Analytics Vidhya and is used on the Writer’s discretion.