Introduction
In a world stuffed with info, PDF paperwork have grow to be a staple for sharing and preserving invaluable information. Nevertheless, extracting insights from PDFs hasn’t all the time been easy. That’s the place “Chat with PDFs” involves the rescue – an revolutionary mission revolutionising how we work together with PDFs.
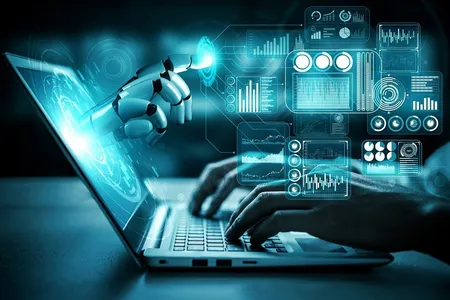
On this article, we introduce you to the fascinating “Chat with PDFs” mission, which mixes the ability of Language Mannequin Libraries (LLMs) and the flexibility of PyPDF’s Python library. This distinctive fusion means that you can have pure conversations along with your PDF paperwork, making it simple to ask questions and get contextually related solutions.
Studying Targets
- Acquire perception into Language Mannequin Libraries (LLMs) as superior AI fashions able to understanding human language patterns and producing significant responses.
- Discover PyPDF, a flexible Python library, to grasp its functionalities for textual content extraction, merging, and splitting in PDF manipulation.
- Acknowledge the mixing of Language Mannequin Libraries (LLMs) and PyPDFs in creating an interactive chatbot for pure conversations with PDFs.
This text was revealed as part of the Knowledge Science Blogathon.
Understanding Language Mannequin Libraries (LLMs)
The guts of “Chat with PDFs” lies in Language Mannequin Libraries (LLMs), superior AI fashions skilled on huge quantities of textual content information. Consider them as language consultants, able to understanding human language patterns and producing significant responses.
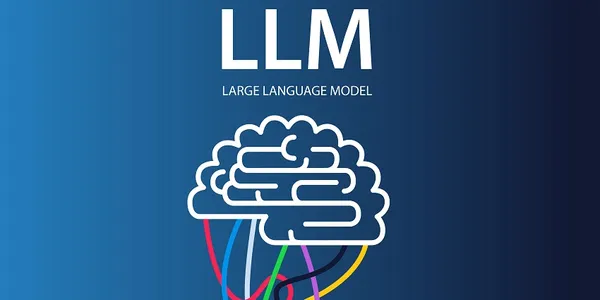
For our mission, LLMs play a significant position in creating an interactive chatbot. This chatbot can course of your questions and perceive what you want from the PDFs. The chatbot can present useful solutions and insights by tapping into the data base hidden in PDFs.
PyPDFs – Your PDF Tremendous Assistant
PyPDF is a flexible Python library that simplifies interactions with PDF information. It equips customers with varied functionalities, akin to textual content extraction, merging, and splitting of PDF paperwork. This library is an important element of our mission, because it allows seamless dealing with of PDFs and streamlines the following evaluation.
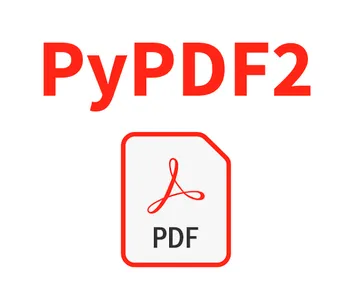
PyPDF helps us load PDF information and extract their textual content inside our mission, setting the stage for environment friendly processing and evaluation. With this highly effective assistant, you possibly can work together with PDFs effortlessly.
Chat with PDFs liberates PDF paperwork from their static state by bringing collectively Language Mannequin Libraries (LLMs) and PyPDFs. Now, you possibly can discover your PDFs like by no means earlier than, extracting invaluable info and interesting in significant conversations. From educational papers to enterprise studies, “Chat with PDFs” makes interacting with PDFs a pleasant expertise.
So, let’s dive into the fascinating world of Chat with PDFs mission.
Venture
# Importing needed libraries and establishing API keys
import os
import pandas as pd
import matplotlib.pyplot as plt
from transformers import GPT2TokenizerFast
from langchain.document_loaders import PyPDFLoader
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.embeddings import OpenAIEmbeddings
from langchain.vectorstores import FAISS
from langchain.chains.question_answering import load_qa_chain
from langchain.llms import OpenAI
from langchain.chains import ConversationalRetrievalChain
from key import openaiapi_key
os.environ["OPENAI_API_KEY"] = openaiapi_key
The code above initiates the “Chat with PDFs” mission by importing important libraries and establishing the API keys. We use the ‘os’ library to work together with the working system, ‘pandas’ for information manipulation, and ‘matplotlib’ for plotting graphs. The ‘transformers’ library gives the ‘GPT2TokenizerFast’ class, which is important for tokenizing textual content. The ‘langchain’ modules embrace courses needed for loading PDFs (‘PyPDFLoader’), textual content splitting (‘RecursiveCharacterTextSplitter’), embeddings (‘OpenAIEmbeddings’), vector storage (‘FAISS’), question-answering chains (‘load_qa_chain’), language fashions (‘OpenAI’), and conversational chains (‘ConversationalRetrievalChain’).
PyPDF Loader
We then use the ‘PyPDFLoader’ class to load the PDF file and cut up it into separate pages. Lastly, we print the primary web page’s content material to confirm the PDF’s profitable loading and splitting.
# Easy methodology - Cut up by pages
loader = PyPDFLoader("story.pdf")
# We're creating an occasion of 'PyPDFLoader' and passing the file path of
#the PDF we need to work with.
pages = loader.load_and_split()
print(pages[0])
This part covers the loading and chunking of the PDF doc. Two strategies are demonstrated: the straightforward methodology that splits the PDF by pages and the superior methodology that entails changing the PDF to textual content and splitting it into smaller chunks.
# Superior methodology - Cut up by chunk
# Step 1: Convert PDF to textual content
import textract
doc = textract.course of("story.pdf")
# Step 2: Save to .txt and reopen (helps forestall points)
with open('story.txt', 'w') as f:
f.write(doc.decode('utf-8'))
with open('story.txt', 'r') as f:
textual content = f.learn()
# Step 3: Create operate to rely tokens
tokenizer = GPT2TokenizerFast.from_pretrained("gpt2")
def count_tokens(textual content: str) -> int:
return len(tokenizer.encode(textual content))
# Step 4: Cut up textual content into chunks
text_splitter = RecursiveCharacterTextSplitter(
# Set a very small chunk measurement, simply to indicate.
chunk_size = 512,
chunk_overlap = 24,
length_function = count_tokens,
)
chunks = text_splitter.create_documents([text])
Steps for Splitting the PDF
The superior methodology splits the PDF into smaller chunks for extra environment friendly processing. We obtain this by way of the next steps:
Step 1: We use the ‘textract’ library to extract textual content from the PDF file and retailer it within the ‘doc’ variable.
Step 2: We save the extracted textual content to a textual content file (‘story.txt’) to stop potential points and reopen it in learn mode. The content material is saved within the ‘textual content’ variable.
Step 3: We outline a operate known as ‘count_tokens’ to rely the variety of tokens in a given textual content. This operate makes use of the ‘GPT2TokenizerFast’ class to tokenize the textual content.
Step 4: Utilizing the ‘RecursiveCharacterTextSplitter’ class, we cut up the ‘textual content’ into smaller ‘chunks’ to make sure environment friendly processing, with every chunk having a most token restrict.
# Embed textual content and retailer embeddings
# Get embedding mannequin
embeddings = OpenAIEmbeddings()
# Create vector database
db = FAISS.from_documents(chunks, embeddings)
OpenAI Embeddings
On this part, we embed the textual content utilizing the ‘OpenAIEmbeddings’ class, which converts textual content into numerical representations (embeddings). These embeddings facilitate environment friendly storage and evaluation of textual information. We then create a vector database utilizing the ‘FAISS’ class, incorporating the ‘chunks’ of textual content and their corresponding embeddings.
# Setup retrieval operate
# Examine similarity search is working
question = "What's the identify of the creator?"
docs = db.similarity_search(question)
docs[0]
Arrange a retrieval operate on this half. You possibly can carry out a similarity search with a pattern question utilizing the vector database (‘db’). The variable question incorporates the query we need to ask the chatbot and the variable docs retailer the related paperwork containing the question’s context. We then print the primary doc returned from the similarity search.
chain = load_qa_chain(OpenAI(temperature=0), chain_type="stuff")
question = "What's the identify of the creator?"
docs = db.similarity_search(question)
chain.run(input_documents=docs, query=question)
On this section, we create a question-answering chain (‘chain’) that integrates the similarity search with person queries. We load the ‘OpenAI’ language mannequin and set the temperature to 0 for deterministic responses. We get hold of a solution primarily based on the data base by passing the retrieved paperwork (‘docs’) and the person’s query (‘question’) to the chain.
# Create chatbot with chat reminiscence
from IPython.show import show
import ipywidgets as widgets
qa = ConversationalRetrievalChain.from_llm(OpenAI(temperature=0.1), db.as_retriever())
chat_history = []
def on_submit(_):
question = input_box.worth
input_box.worth = ""
if question.decrease() == 'exit':
print("Thanks for utilizing the Chat with PDFs chatbot!")
return
end result = qa({"query": question, "chat_history": chat_history})
chat_history.append((question, end result['answer']))
show(widgets.HTML(f'<b>Person:</b> {question}'))
show(widgets.HTML(f'<b><font shade="blue">Chatbot:</font></b>{end result["answer"]}'))
print("Welcome to the Chat with PDFs chatbot! Kind 'exit' to cease.")
input_box = widgets.Textual content(placeholder="Please enter your query:")
input_box.on_submit(on_submit)
show(input_box)
Within the closing part, we introduce a chatbot characteristic the place Customers can work together with the chatbot by coming into questions and get solutions.
Conclusion
This text explored the fascinating “Chat with PDFs” mission and its step-by-step implementation. We’ve gained a deeper understanding of Language Mannequin Libraries (LLMs) and PyPDFs, two important elements powering this revolutionary software. Now you possibly can effortlessly course of and analyze PDF paperwork, extracting invaluable insights and interesting in interactive conversations with a chatbot companion. Whether or not you’re a researcher, scholar, or skilled, “Chat with PDFs” has revolutionized how we work together with PDFs, making the beforehand static paperwork come to life with the ability of AI. Pleased PDF exploring!
Key Takeaways
- LLMs empower the chatbot to ship correct and context-aware responses to person queries.
- PyPDF simplifies PDF manipulation, making it simpler to work with advanced paperwork.
- The code’s construction ensures clean integration of textual content embedding and similarity search functionalities.
- PyPDF allows seamless PDF dealing with, textual content extraction, and manipulation.
Incessantly Requested Questions
A. Massive Language Fashions are highly effective AI fashions skilled on huge quantities of textual content information. They’ll perceive human language and carry out varied pure language processing duties, akin to textual content technology, summarization, and question-answering.
A. LLMs create a chatbot that may reply person queries primarily based on info extracted from PDF paperwork. The LLM processes and understands person questions and gives related responses from the data base.
A. PyPDF is a Python library designed for working with PDF information. It allows duties like textual content extraction, merging, and splitting of PDF paperwork, making it a invaluable software for PDF-related duties in a programmatic method.
A. PyPDF hundreds pdf information and extracts their content material into pdfs. The extracted textual content is then processed and cut up into smaller chunks, facilitating environment friendly processing and evaluation.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Creator’s discretion.