Beginning with iOS 16, SwiftUI introduces a local photograph picker view often called PhotosPicker
. In case your app requires entry to customers’ photograph library, the PhotosPicker view seamlessly manages the photograph choice course of. This built-in view gives outstanding simplicity, permitting builders to current the picker and deal with picture choice with just some strains of code.
When presenting the PhotosPicker
view, it showcases the photograph album in a separate sheet, rendered atop your app’s interface. In earlier variations of iOS, you couldn’t customise or change the looks of the images picker view to align along with your app’s structure. Nonetheless, Apple has launched enhancements to the PhotosPicker view in iOS 17, enabling builders to seamlessly embed it inline inside the app. Moreover, you’ve gotten the choice to switch its dimension and structure utilizing normal SwiftUI modifiers similar to .body
and .padding
.
On this tutorial, I’ll present you learn how to implement an inline photograph picker with the improved PhotosPicker
view.
Revisiting Picture Pickers
To make use of the PhotosPicker
view, you possibly can first declare a state variable to retailer the photograph choice after which instantiate a PhotosPicker
view by passing the binding to the state variable. Right here is an instance:
struct ContentView: View {
@State non-public var selectedItem: PhotosPickerItem?
var physique: some View {
PhotosPicker(choice: $selectedItem,
matching: .photographs) {
Label(“Choose a photograph”, systemImage: “photograph”)
}
}
}
import SwiftUI import PhotosUI Â struct ContentView: View { Â Â Â Â Â @State non-public var selectedItem: PhotosPickerItem? Â Â Â Â Â var physique: some View { Â Â Â Â Â Â Â Â PhotosPicker(choice: $selectedItem, Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â matching: .photographs) { Â Â Â Â Â Â Â Â Â Â Â Â Label(“Choose a photograph”, systemImage: “photograph”) Â Â Â Â Â Â Â Â } Â Â Â Â } } |
The matching
parameter lets you specify the asset sort to show. Right here, we simply select to show photographs solely. Within the closure, we create a easy button with the Label
view.
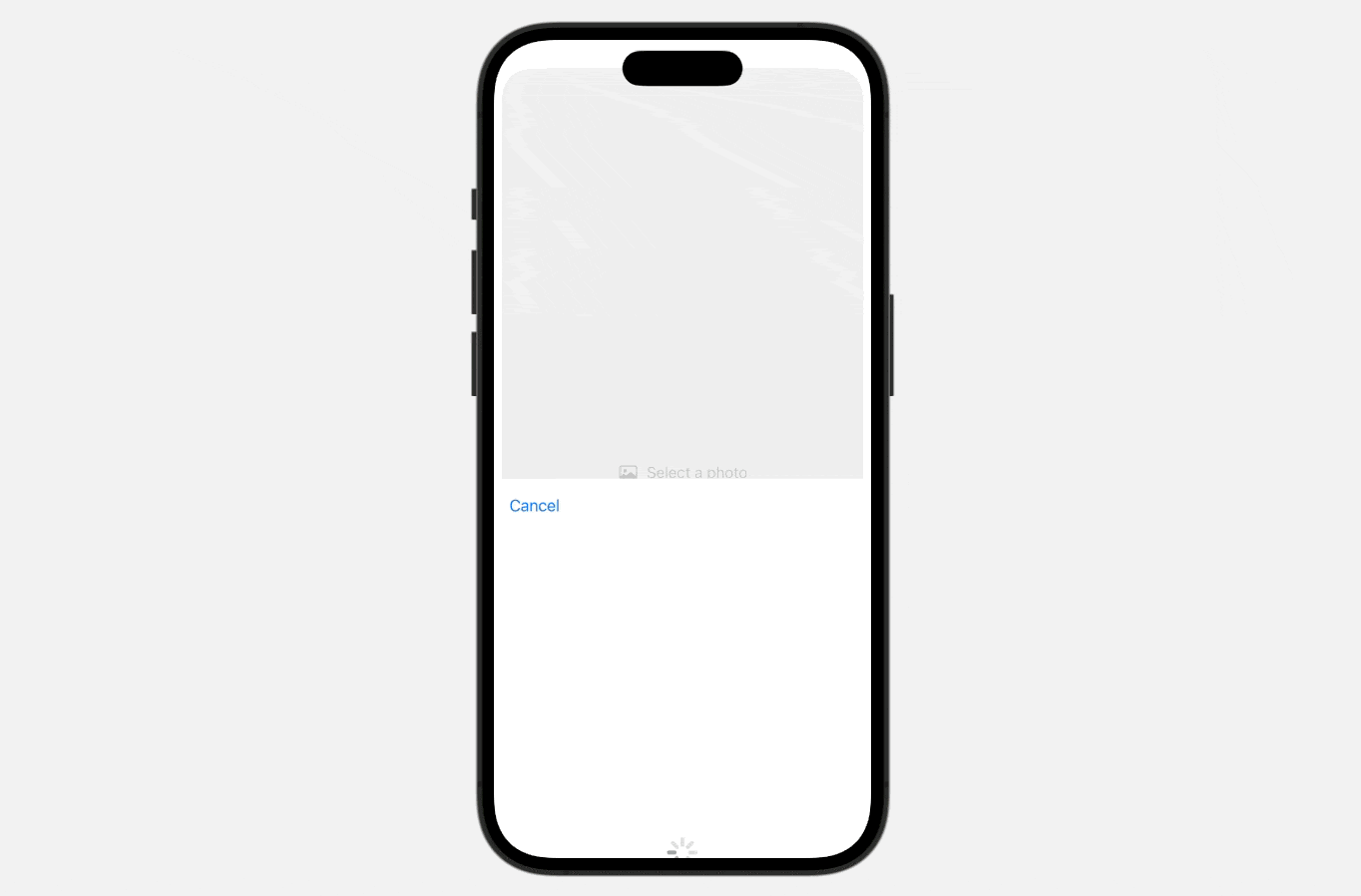
Upon choosing a photograph, the photograph picker mechanically dismisses itself, and the chosen photograph merchandise is saved within the selectedItem
 variable, which is of sort PhotosPickerItem
. To load the picture from the merchandise, you should use loadTransferable(sort:completionHandler:)
. You possibly can connect the onChange
 modifier to take heed to the replace of the selectedItem
variable. At any time when there’s a change, you name the loadTransferable
 methodology to load the asset knowledge like this:
.
.
.
.onChange(of: selectedItem) { oldItem, newItem in
Activity {
if let picture = strive? await newItem?.loadTransferable(sort: Picture.self) {
selectedImage = picture
}
}
}
@State non-public var selectedImage: Picture?  . . .  .onChange(of: selectedItem) { oldItem, newItem in     Activity {         if let picture = strive? await newItem?.loadTransferable(sort: Picture.self) {             selectedImage = picture         }     } } |
When utilizing loadTransferable
, it’s essential to specify the asset sort for retrieval. On this case, we make use of the Picture
 sort to straight load the picture. If the operation is profitable, the tactic will return an Picture
 view, which can be utilized to straight render the photograph on the display.
if let selectedImage {     selectedImage         .resizable()         .scaledToFit()         .padding(.horizontal, 10) } |
Implementing an Inline PhotosPicker
Now that it’s best to perceive learn how to work with a PhotosPicker
, let’s see learn how to embed it in our demo app. What we’re going to do is to exchange the “Choose a photograph” button with an inline Photographs picker. The up to date model of PhotosPicker
comes with a brand new modifier referred to as photosPickerStyle
. By specify a price of .inline
, the Photographs picker might be mechanically embedded within the app:
.photosPickerStyle(.inline) |
It’s also possible to connect normal modifiers like .body
and .padding
to regulate the dimensions of the picker.

By default, the highest accent of the picker is the navigation bar and the underside accent is the toolbar. To disable each bars, you possibly can apply the photosPickerAccessoryVisibility
modifier:
.photosPickerAccessoryVisibility(.hidden) |
Optionally, you possibly can conceal both of them:
.photosPickerAccessoryVisibility(.hidden, edges: .backside) |
Dealing with A number of Picture Alternatives
Presently, the Photographs picker solely permits customers to pick out a single photograph. To allow a number of picks, you possibly can decide within the steady choice habits by setting the selectionBehavior
to .steady
or .continuousAndOrdered
:
PhotosPicker(choice: $selectedItems, Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â maxSelectionCount: 5, Â Â Â Â Â Â Â Â Â Â Â Â selectionBehavior: .continuousAndOrdered, Â Â Â Â Â Â Â Â Â Â Â Â matching: .photographs) { Â Â Â Â Label(“Choose a photograph”, systemImage: “photograph”) } |
In case you want to limit the variety of objects accessible for choice, you possibly can specify the utmost depend utilizing the maxSelectionCount
 parameter.
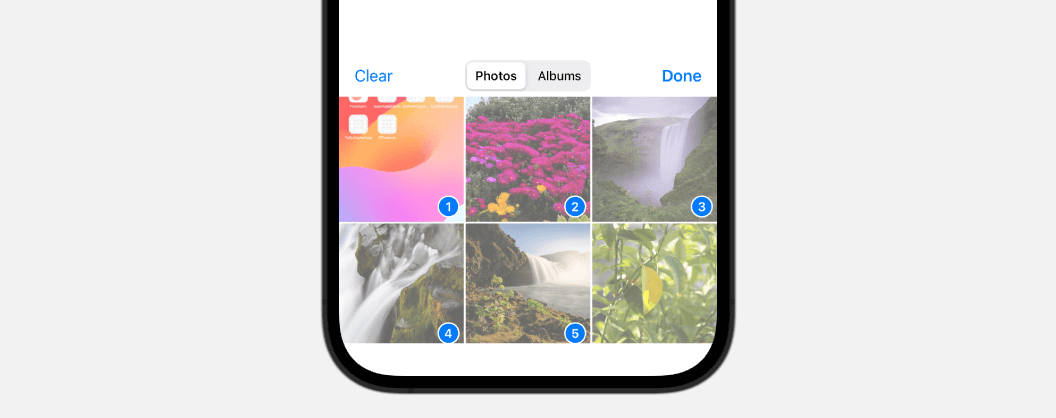
As soon as the consumer has chosen a set of images, they’re saved within the selectedItems
 array. The selectedItems
 array has been modified to accommodate a number of objects and is now of sort PhotosPickerItem
.
@State non-public var selectedItems: [PhotosPickerItem] = [] |
To load the chosen images, you possibly can replace the onChange
closure like this:
selectedImages.removeAll()
newItems.forEach { newItem in
Activity {
if let picture = strive? await newItem.loadTransferable(sort: Picture.self) {
selectedImages.append(picture)
}
}
}
}
.onChange(of: selectedItems) { oldItems, newItems in      selectedImages.removeAll()      newItems.forEach { newItem in          Activity {             if let picture = strive? await newItem.loadTransferable(sort: Picture.self) {                 selectedImages.append(picture)             }         }      } } |
I used an Picture
array to retailer the retrieved photographs.
@State non-public var selectedImages: [Image] = [] |
To show the chosen photographs, chances are you’ll use a horizontal scroll view. Right here is the pattern code that may be positioned in the beginning of the VStack
view:
ScrollView(.horizontal) {
LazyHStack {
ForEach(0..<selectedImages.depend, id: .self) { index in
selectedImages[index]
.resizable()
.scaledToFill()
.body(top: 250)
.clipShape(RoundedRectangle(cornerRadius: 25.0))
.padding(.horizontal, 20)
.containerRelativeFrame(.horizontal)
}
}
}
.body(top: 300)
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
if selectedImages.isEmpty {     ContentUnavailableView(“No Photographs”, systemImage: “photograph.on.rectangle”, description: Textual content(“To get began, choose some images under”))         .body(top: 300) } else {      ScrollView(.horizontal) {         LazyHStack {             ForEach(0..<selectedImages.depend, id: .self) { index in                 selectedImages[index]                     .resizable()                     .scaledToFill()                     .body(top: 250)                     .clipShape(RoundedRectangle(cornerRadius: 25.0))                     .padding(.horizontal, 20)                     .containerRelativeFrame(.horizontal)             }          }     }     .body(top: 300) } |
In case you’d wish to be taught extra about learn how to create picture carousels, you possibly can try this tutorial. In iOS 17, a brand new view referred to as ContentUnavailableView
 is launched. This view is really helpful to be used in situations the place the content material of a view can’t be displayed. So, when no photograph is chosen, we use the ContentUnavailableView
 to current a concise and informative message.
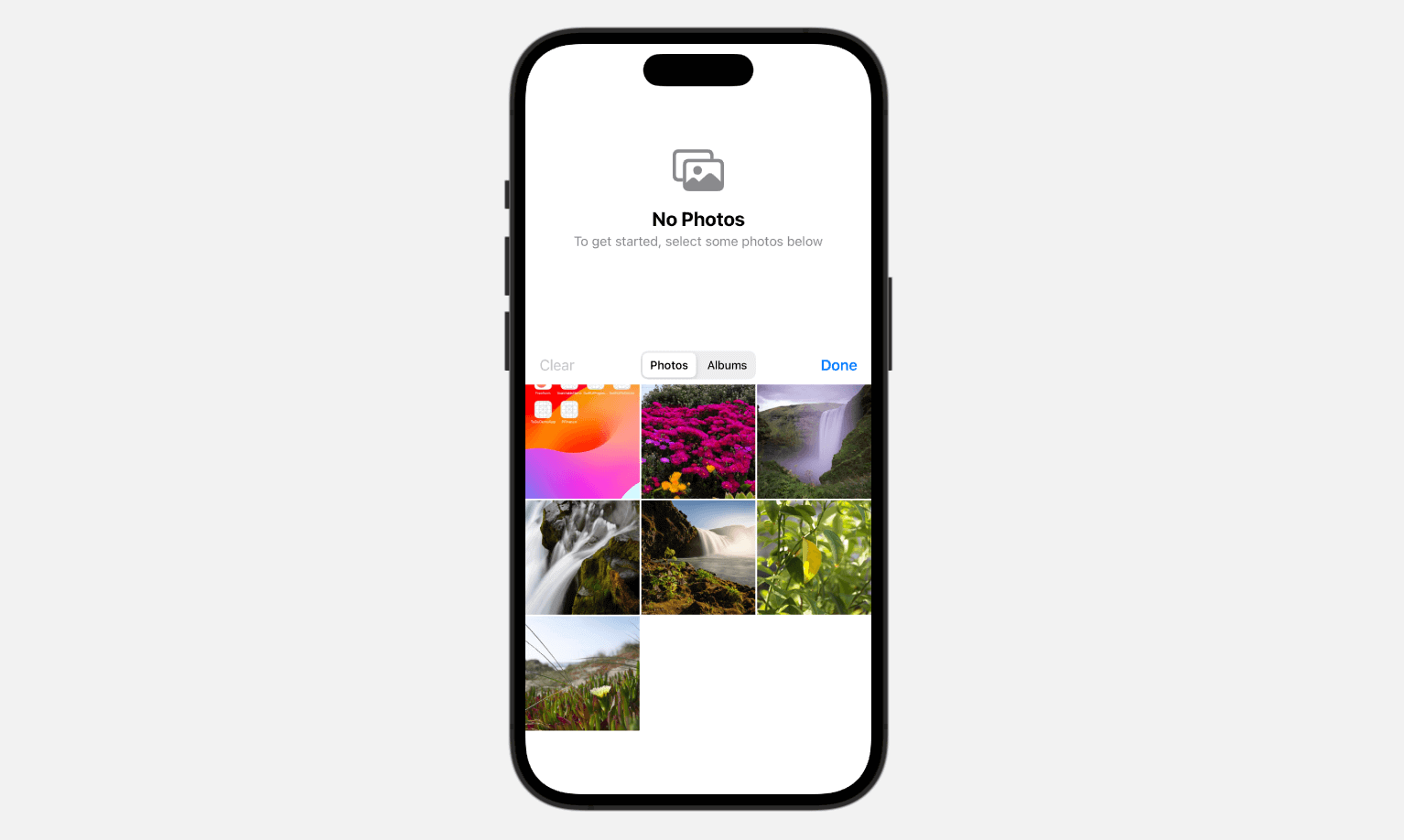
Abstract
In iOS 17, Apple made enhancements to the native Photographs picker. Now, you possibly can simply embrace it inside your app as an alternative of utilizing a separate sheet. This tutorial explains the brand new modifiers that include the up to date PhotosPicker
view and exhibits you learn how to create an inline photograph picker.
In case you take pleasure in studying this tutorial and wish to dive deeper into SwiftUI, you possibly can try our Mastering SwiftUI e book.